HTML, CSS & JavaScript: The Basics Part I
Becoming a proficient web developer is hard — but understanding the basics isn’t. By the end of this tutorial, you should have an idea of what people mean when they talk about HTML, CSS and JavaScript.
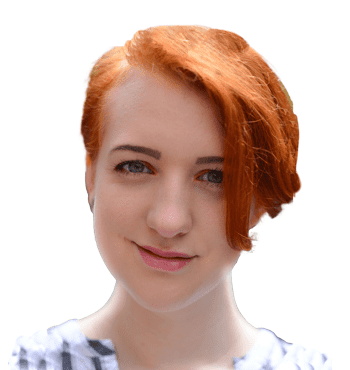
HTML, CSS and JavaScript are what most webpages are made of. They work together, each with a specific role. While you write a webpage, it will basically just look like a text document. You could write it in any text editor of your choice, although it’s probably a good idea to use a code editor like Atom or Sublime Text that will help you with formatting and code completion as soon as you tell it what's the language you've written it in, for example by saving the file with the ending .html.
Once you’ve written the page, your browser is where the magic happens. It interprets the code you wrote in your .html, .css and .js text files and constructs a fully functioning, beautiful website from it – given there’s no errors in your code, of course. But that’s what you’re here for today.
HTML, or HyperText Markup Language, is the backbone of any webpage. It determines its basic structure and content. However, if you only use HTML, your webpage will end up looking like the very first pages from the early days of the web. No pretty formatting, no interactive elements. You’ll see it sometimes when your internet connection is very slow and your browser won’t load the page properly. It’s okay, but we can to better. That’s what CSS is for.
CSS is short for Cascading Style Sheets. This is the code that tells your browser how your webpage should look: What fonts and colors to use, what size your text should be etc.. But a pretty-looking website is not all you could want. You might want to interact with the webpage, click things, move stuff around and have it respond to your actions. HTML and CSS can’t do that very well, but JavaScript can.
JavaScript tells your browser how the page should behave. It’s what you write interactive graphics with, or pretty much any interactive element on your website. Compared to the other two, it’s a pretty complex language that you probably won’t learn entirely in a week or two. But that’s okay. For now, it’s enough to know what it does
So if you only remember one thing from this tutorial, let it be this summary of what makes up a webpage:
HTML: Strucure/content
CSS: Style
JavaScript: Behaviour
If your mind has a little more room left right now, then let’s take a closer look at how to build a webpage. As mentioned, webpages are nothing but text documents that your browser knows how to interpret. To write these documents, you can use any code editor of your choice. I use Atom, which works very nicely as long as you don’t try to view giant datasets.
We’re going to look at some useful commands in this tutorial. But of course, there’s lots more to discover. If you want to look up more stuff, W3schools is a good place to start. You’ll find references for HTML, CSS, JavaScript and more. There are also some good MOOCs (Massive Open Online Courses) for web development. Take a look over at Coursera or CodeAcademy, for example.
It’s always a good idea to look at the code of other webpages and see how they work. To do so, right click anywhere on a website. The details are different for each browser, but there will be an option like “Inspect element” and “Show source code”. If you click it, you should see a lot of code. This is the website how your browser sees it. Try to understand what’s going on. This is a good source of inspiration for your own pages as well.
While coding your own projects, it might also be able to take a look at the console of your browser. You can find it by clicking “Inspect element” (or whatever corresponds to that in your browser) and choosing the tab named “Console” in the window that opens. The console will show you an error message if something isn’t right with your page. That message can help you identify the problem, or at least give you something to google if you don’t understand the problem right away.
But you’ll get to that later. First, let’s get to know the basics.
HTML
Let’s start with some HTML code. If you want to code along, open up a new document in the code editor of your choice. Copy the code below into the file and save it as, for example, index.html (the .html part is the important one).
<!DOCTYPE html>
<html>
<head>
<title>My webpage</title>
<style> <!--Some CSS--> </style>
<script> <!--Some JavaScript--> </script>
</head>
<body>
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
</body>
</html>
This is the structure each HTML document follows. The first thing you’ll notice is lots of angle bracket action. The words in between the angle brackets are keywords we call tag names. Together with the bracket, they make up a tag that serves a specific function. HTML content is always placed between a start tag and an end tag that begins with a slash, like this:
<tagname>content</tagname>
Most tags accept attributes that specify how they should behave:
<tagname arg1 = value1 arg2=value2>content</tagname>
We’ll get to know some examples of that soon.
Any HTML document starts with the declaration <!DOCTYPE html> that tells the browser what kind of document to expect (html in this case, duh). Then, there’s the <html> tag. It doesn’t close until the very end of the page and makes it extra clear that anything in between will, in fact, be HTML code.
The two basic parts of your page are the <head> and the <body>. The head contains all the meta information you want or need to make your website function properly. It can be used, for example, to give your website a name (<title>), load necessary CSS information (<style>) or JavaScript sources (<script>), which we’ll get to later on. You can also set lots of other stuff like the language or the character encoding in the head.
Anything in the head will not be directly visible on the webpage, so if you want to finally add some content, take a look at the body of your page. This is where you put your actual content. There’s quite a few options as to how to write your text and what media to incorporate into your page. Let’s take a quick look at a few important tags for that. If you want, copy them into your freshly made HTML document and play around with them a little. To see what they do, just save the file and open it in your browser (you can leave it open in your code editor as well, of course).
Formatting
- <p> stands for “paragraph”. Use it to write some important stuff onto your website, and don’t forget to close your paragraph with </p>. End tags are important for almost all the tags we’ll discuss here.
- <h1> ... <h6> are headings. The higher the number, the smaller the font of your heading will be.
- <b> or <strong> ist used to use bold print like this for your text. Both commands do essentially the same thing.
- <i> or <em> are both used to write in cursive.
- <u> underlines text, but you can forget about that one instantly. On the web, it’s pretty much never a good idea to underline text that’s not a link, and those are underlined by default.
- <br> creates a line break. This is one of very few so-called void tags that don’t need a closing tag.
Lists
- <ul> creates an unordered list like the one you’re reading right now.
- <ol> creates an ordered list. It can be modified with some attributes. For example, <ol start="343" reversed> will start the list at number 343 instead of 1 and count down from there instead of up. Here you can find a list of all possible attributes.
- <li> creates a list element to populate the unordered or ordered lists you created.
- Your shopping list in HTML might look something like this:
<ul> <li>Coffee</li> <li>Tea</li> <li>Milk</li> </ul>
Media
- Links: <a href="URL" target="_blank">Link text</a> The “a” stands for anchor, and is used to create a link. Put the URL you want to refer to in the href attribute. target=”_blank” makes the link open in a new tab. If you leave it out, it will default to opening the link in the current tab.
- Images: <img scr="file path/URL" height="550" width="100%"> The img tag is another void tag, so you don’t need to type </img> after it. Try experimenting with the width and height settings. If you specify them as just a number, the unit will default to pixels. By specifying percentages, you can scale the image according to the screen it’s viewed on. With the above specifications, for example, our picture will the entire width of our screen and be 550 pixels high. Note that if you specify both width and height, you might skew the proportions of your image. That is why sometimes, it’s better to only specify either the width or the height settings.
- Audio: <audio src="file path/URL" controls autoplay loop> Sorry, your browser can't handle this </audio> The audio tag lets you incorporate any audio file into your website. It’s probably a good idea to type in controls as an attribute so the user can pause and unpause the audio. Autoplay and loop are pretty self-explanatory. Better think twice about whether you actually want your audio to play instantly and on a loop, though. The text between the opening and closing tag of this line is only displayed if the browser can’t load the audio file to inform users that there should, in fact, be something here.
- Video: <video src="file path/URL" controls autoplay loop> Sorry, your browser can't handle this </video> The video tag works pretty much the same as the audio tag, just, well, with videos. You can also specify width and height like with an image.
Inline Frames
<iframe src=“file path/URL“ height=“550“ width=“100%“ scrolling style=„border:none“>
Sorry, your browser can’t handle this
</iframe>
An Inline frame, or iframe for short, works pretty much like the video ot audio tags, but it’s much more powerful. It let’s you display any content, even a whole other website, inside the frame you specify. It’s kind of like a window to another page. It’s very useful for easily displaying graphics or web apps and such, but it can be a bit tricky to make it properly responsive. So if you’ve got the chance, always try to natively integrate your pretty interactive graphics into your webpage. But if you don’t have the time or resources, an iframe will do just fine. Take a look at its possibly attributes here.
Structure
There’s some HTML tags that don’t appear to be doing anything on first sight. They’re structural elements designed to let you style the layout of your page.
- <div>content</div> A div, short for division, is one of those elements. It’s used to define a separate section in your HTML document. If you don’t use CSS or JavaScript to tell the browser what to do with it, you won’t even notice it’s there. But if used properly, divs can be a great layout tool.
- A <span> is like the divs little sibling. While a div can be used to style entire sections of a text, move them or give them a specific format, <span> is more commonly used for styling smaller elements, like if you want to give part of your sentence a different color.
If you’ve followed this tutorial and tried out some of the options we’ve discussed so far, you should have something that resembles a website from the early 2000s by now. That’s pretty cool. Let’s see if we can make it even cooler. If you want to learn about styling your website with CSS and making it interactive with JavaScript, please continue with part two of our tutorial. See you there!
Credits for the awesome featured image go to Phil Ninh